- Game Pigeon 20 Questions Answers Questions And Answers
- Game Pigeon 20 Questions Answers Answer
- Game Pigeon 20 Questions Cheats
- Game Pigeon 20 Questions Answers Multiple Choice
- Game Pigeon 20 Questions Answers Questions
In Palmer's dream, what does a pigeon's eye look like? A shirt button, orange with a black dot in the center. Who has Palmer invited to his birthday party? Beans, Mutto and Henry. How does Palmer feel when the boys show up to his party? He feels he is finally accepted into their group. (read all 180 Short Answer Questions and Answers).
0. Background
There are many variations of the well-known '20 Questions Game'. But the basic rules are straightforward: You're at least two players, one asks a question and the other player answers. There are no right or wrong answers. The main goal of the game isn't even answering a question, it's sharing and talking about personal things. Pigeonetics Game Teacher Guide Pigeonetics Game - Teacher Guide 1 Learn.Genetics.utah.edu GENETIC SCIENCE LEARNING CENTER Breed pigeons for desired traits while learning the basic laws of inheritance. Choose pigeons with the right genotype, and breed them to yield offspring with a specified phenotype in 26 puzzles of increasing complexity.
A. Goals
The goal of this assignment is to provide a practical use of searching and sorting, while simultaneously building up familiarity with 2D arrays. In this assignment, you will build a modified version of the game 20 Questions. By the end, you will be familiar with:
- 2D arrays,
- selection and insertion sort conceptually and their implementations, and
- understanding how recursion is used to search for specific elements in a dataset.
B. Background
As a child, you may have played a game called '20 questions', in which one player thinks of an animal, person, or object and the other person guesses the secret item by asking 20 questions. In this assignment you will be writing a modified version of this game. Your program will ask you to think of an animal, and then ask you up to N
yes or no questions in order to guess the animal you're thinking of. The questions and possible outcomes will be determined through a dataset we provide.
An online example of the game 20 Questions can be found at www.20q.net.
C. Your Program
First, download the skeleton code for GuessingGame.java
, the data sets animals_small.txt
and animals.txt
, and the library Prompter.java
for getting interactive input.
By the end of the assignment, your program GuessingGame
will (1) read in questions and answers data set from a file, (2) store the data in a 2D array, (3) sort the data so it can be easily searched, and (4) interact with the user to search for a target item. It will prompt the user with a number of questions until a single answer can be decided on. If there is no match the program should let the user know its been stumped.
The final product will look like:
1. Reading the Data
A. Data Format
Before you can start asking and answering questions, your program will need to have a large set of animals and some information about them. To do this, you'll read in a file in the following format:
animals_small.txt:
First, it provides the number of animals and questions in the data set. Then all the questions, each on their own line, and finally a list of animals and the answers to the questions ('y' is for yes; 'n' is for no). For instance, if you look at the line owl n y n
, the first column is the name of the animal (owl), the second is the answer to the first question (an owl is not a mammal), the third to the answer to the second question (owls are carnivores), and the last is the answer to the third question (owls do not stand on four legs). Once you feel comfortable with the format of the data, move on to the next section.
B. readData()
Now that you know what format the data will be in, you will write the function, readData(String filename)
, to parse through the animal.txt
file.
readData()
should be doing two things: 1.) initializing and populating a class-level array called String[] questions
, and 2.) initializing and populating a class-level 2D array String[][] answers
. Note that in the skeleton code we provided, readData()
has a void
return type, so you should not be returning either questions
or answers
.
After running readData('animals_small.txt')
, your arrays should contain:
Note that each row (the first index) in answers
corresponds to an animal, and each column (the second index) corresponds to an answer to a question (or the animal's name). Be careful -- it is very easy to mix this up. For reference, you can access a particular animal's attribute via answers[animalIndex][questionIndex]
, where animalIndex
and questionIndex
are the indices of the animal and question respectively (write this down somewhere!).
If you need a reminder how to read in from files, look back at previous homework assignments and read about In inStream
. Once you believe your readData()
is correct by eye, write the print()
function in the next section and then test your two functions in main()
.
C. print()
Being able to easily debug is essential when writing large programs. With this in mind, write a print()
function that outputs the content of the two class-level arrays.
print()
should print 1.) the number of animals, 2.) the number of questions, 3.) the questions
array, and 4.) the answers
array. The output should look EXACTLY like the file you read in, but you should construct this output based solely on the questions
and answers
arrays. Be sure that each animal has all its info on the same line in your output, and compare it to the original file.
To check whether your program is correct so far, write a short main()
function to first call readData('animals_small.txt')
and then print()
. Make sure that the output is identical to the content of the animals_small.txt
file. Once you can successfully do this, move on to the next section.
2. Sorting the Data
The next step is to sort the data set so that we can use standard searching techniques efficiently. However, unlike the sorting code we covered in class, we now have to deal with the 2D structure of the array AND the fact that we will be asking multiple questions.
Essentially, we need to reorder the rows (i.e., animals) so that each column is in sorted order, subject to row-order of the previous questions.
This complex idea should be easier to understand with an example. Imagine that we have the following small data set
We must first re-order the rows so that the first column is in sorted order:
This effectively divides the data set into two parts (blue and red), depending on the answer to the first question. Then, we have to re-order the rows WITHIN the blue and red blocks such that the 2nd column is in order:
Now, we effectively have four blocks, corresponding to the values of the first two attributes (nn, ny, yn, yy):
Next, we must sort the rows WITHIN each of these four blocks by the third column, yielding:
Notice that we reorder the rows WITHIN each block, but NEVER ACROSS blocks. We would then keep repeating this process for all subsequent attributes.
Read this example over several times until you thoroughly understand it. Once you do understand conceptually how this sort must work, move onto the next section.
B. Sorting in sort()
To do this sort, you will write several functions.
The first function you should write is:
public static void sortRange(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
This should sort the rows in the 2D array answers
from row indices startingAnimalIdx
to endingAnimalIdx
(not including endingAnimalIdx
) based on the column at index questionIdx
. For instance, if answers
looks like
and you called sortRange(0, 2, 2)
, you would be sorting the first two rows by the second column:
We can see that 'n' is earlier in the alphabet than 'y', and so these two rows should be switched, resulting in:
You are free to write this sorting function using either insertion sort or selection sort. You can look at the lecture materials to see a code implementation of either sort for 1D arrays, and you can base your code off the lecture code. However, not that your code will be substantially different from the lecture code since it must deal with the 2D structure of the array.
For comparing values, you should be using the String.compareTo()
function to compare the Strings. With Strings a
and b
, a.compareTo(b)
will return a negative number if a < b
, positive if a > b
, and zero if they are equal.
Once you can sort a column within a particular range of rows, you can move on to the next section. Write some code in main() to test your implementation. Remember, if you are having trouble debugging, use the print()
function to help figure out what is going wrong! Make sure it works correctly before moving onto the next part.
C. Finding the Split
We have one more helper function to write before we can do a complete sort through the data set. For every question, once we use sortRange()
to sort answers
by a question
, there will be an animal where everything above it answers 'n'
to question
and everything below has answers 'y'
. You will want to write the function
public static int findSplit(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
that will find the index of the first animal row that has the answer 'y'
to the question questionIdx
within the range startingAnimalIdx
to endingAnimalIdx
(not including endingAnimalIdx
). Note that this is a bit different than the standard binary search we discussed in class.
You should be comfortable with the intuition of binary search before you start coding!
Remember you will want to find the midpoint, and depending on the answer to the questionIdx
at that row, you will want to recurse either to the top or bottom half of the search range. You will need to check the value immediately below the midpoint in order to determine if it is the first 'y' to appear. If all of the answers in the range are 'y' or all are 'n', you'll want to return -1
.
Once you have this, you should be able to test it on animals_small.txt
. For example, findSplit(0, 4, 1)
should return 2.
If you are unable to get binary search working to find the split point, you may use linear search for partial credit.
D. Recursing in sort()
Once sortRange()
and findSplit()
are done, we then want to sort the entire answers
array, starting at the first question and then moving onto subsequent questions, exactly as in the example at the top of this section. This will be done recursively, which means writing a base case and recursive calls within the sorting code.
Write a recursive helper function public static void sort(int startingAnimalIdx, int endingAnimalIdx, int startingQuestionIdx)
to implement this sorting process. Note that this has a very similar signature to sortRange()
but behaves very differently in one respect -- this function sorts the given range of animals COMPLETELY, starting with the column index startingQuestionId
and moving onto all SUBSEQUENT columns. (In contrast, sortRange()
only sorts the given range for that particular column.)
Base Case: The base case is if the question
you are sorting by does not exist (i.e., you only have 3 questions but you are trying to sort based on the 4th question) OR if there is only one row remaining in the given range. In this case, you simply want to return without doing anything.
Recursive Calls: For each column, you'll want to make two recursive calls. Say, in animals_small.txt
you have split the group into mammals and non-mammals:
Next you want to look at only the non-mammals and split it by whether they are carnivores, then look only at the mammals and split by whether they are carnivores.
Following that intuition, we will want to do two recursive calls for every column, one for the 'n's, and one for the 'y's. So for each, you should find the top and bottom of the range to pass as the startingAnimalIdx
and endingAnimalIdx
arguments to sort()
(Note: this is where findSplit
comes in!). Remember to deal with the edge case where findSplit
returns -1
. Within the mammals, you don't want to sort by the mammals question again, so your question
argument shouldn't be the same, but should move to the next question (question + 1
).
Once you have finished and tested this recursive helper function, you should go back implement the sort()
function to recursively sort all columns of answers
. Think about what the initial range of the sort should be, and the first question that it should be sorted by.
3. Searching the Data
A. Narrowing the Possibilities
Now that we have our data ordered, we can quickly search through it. Consider the animals in animals_small.txt
:
Before your first question, all you know is that your search range is the entire list of animals. Once you ask the first question, and know that the animal is a mammal, your search range suddenly gets chopped in half:
This process is very similar to the binary search you talked about in lecture and implemented in findSplit
, although the split won't always be perfectly in half.
In order to make GuessingGame
interactive, we've provided the Prompter.java
library. It has only one function:
String prompt(String prefix)
If you call Prompter.prompt('> ')
it will print '> '
, wait for the user to enter a String
, and then returns that String
.
Once you feel comfortable with the conceptual side of this search, move on to write search()
.
B. search()
You now have all the pieces in place to begin guessing some animals! Similarly to sort()
, you will see there are two functions in your skeleton code called search
.
First, let's look at:
public static String search(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
This function should search through your data set, considering only the range from startingAnimalIdx
to endingAnimalIdx
, looking first at questionIdx
. As in other functions, animals[endingAnimalIdx]
is not included in the search range. It will be structured as a binary search. If you'd like a refresher, you should look back a the lecture material on binary search (there is even a code implementation there!).
search()
will be recursive, so you will need a base case and recursive calls:
Base Case: The base case is when there is only one element in your search range. Note that the answers[endingAnimalIdx]
row is not in the search range. You should return the single element's name (the actual name of the animal found in the zeroth column).
Function Body: You should System.out.println
the question
text and then use Prompter.prompt('> ')
to get the answer.
Recursive Call: First find the 'n' and 'y' split using findSplit
, then, if the user answered 'n', search from the startingAnimalIdx
of the range to where the split is. If the user answered 'y', search from the split to the endingAnimalIdx
of the range. You will also want to move on to the next question (question + 1
).
Once you have finished this function, complete:
public static String search()
It should be only one line long and call search(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
and search the entire list starting with the first question.
If this is done correctly, you should be able to play the game with the following code in your main()
:
4. Extra Credit
Game Pigeon 20 Questions Answers Questions And Answers
A. Create Your Own Dataset!
For extra credit, you can collect your own dataset to query using your program! Make sure it adheres to these rules:
- No two items should have the same set of answers. For instance, a bear and a lion are both carnivorous mammals that stand on four feet, so with the questions in
animals_small.txt
we wouldn't be able to differentiate them. - All answers should be 'yes' or 'no', and so all questions should be yes-no questions.
- The data set should contain at least 50 items
Before you submit, make sure your dataset works with your Program!
5. Submission
A. README
Complete readme_guessing.txt
in the same way you have done for previous assignments.
Game Pigeon 20 Questions Answers Answer
B. Submission
Submit GuessingGame.java
and readme_guessing.txt
on the course website. If you have completed extra credit please mention this in the readme, and submit it compressed as extra.zip
.
Before submission remove any print statements that were used for debugging or testing your functions.
Be sure that every method has an appropriate header comment, and that your code is well-documented.
To challenge your friends within iMessage:
Game Pigeon 20 Questions Cheats
- Enter a conversation with the person you want to play with.
- Tap the Apps icon located next to the iMessage text box.
- From the Apps menu, tap the Grid icon in the bottom-left of the screen.
- Scroll through your apps to find the game you want to play and tap it.
- Tap Create Game.
How do I get iMessage games on my iPhone?
Tap on '>' and tap on the App Store icon. Step 3. Tap the square dot icon and find the game you just installed in iMessage. Step 4.
How do you play games on iMessage?
Getting started with iMessage games is easy. First, bring up the conversation with your friend. Then select the App Store icon in the bar below the message box. That will bring up the iMessage App Store with games, stickers, and more for use only in the Messages app.
Can Android Play iMessage games?
The iMessages need to be sent through Apple's servers, and the only way to do this legitimately is to use an Apple device. Using an app running on a Mac computer as a server that relays messages to the Android device is a very smart way to make iMessage work on Android, where it is not technically supported.
How do you play games on iPhone?
Before you can play any game on the Messages app on your iPhone, you need to download the games from the App Store in Messages. To do that, follow these steps: 1.Go to your Home screen by pressing your iPhone's Home button. 2.From the Home screen, open your Messages app.
What are iMessage games?
There are three types of iMessage Apps you can install — games, apps, and stickers. You can access the iMessage App Store from the Messages app by tapping the App Store icon near the keyboard in a conversation. The list of stickers, games, and apps for iMessage are continuing to grow, and plenty more will come.
How do I get iMessage?
To use iMessage or FaceTime, you need to activate them on your iPhone, iPad, or iPod touch.
Turn off and restart iMessage and FaceTime
- Go to Settings > Messages and turn off iMessage.
- Go to Settings > FaceTime and turn off FaceTime.
- Restart your device.
- Turn iMessage and FaceTime back on.
Can Apple make iMessages on Android?
Apple May Make iMessage Work with Android (Report) Google already supports RCS in its Android Messages app, but so far only Sprint among the major U.S. carriers supports the protocol.
Is there an Android version of iMessage?
iMessage is so good that many smartphone users would love to see an Android version come out, although it's something Apple will probably never do. Android Messages, not to be confused with Hangouts or Allo, is Google's texting app, and a new version of the app will soon be available on your Android device.
Can you play iMessage games on iPad?
Open the Message App on your iPhone/iPad and enter a thread. Then tap on the App Store icon and you can visit App Store for iMessage to explore games on your device. In App Store, you can install games you want that are compatible to iMessage.
How do you play games on messenger?
How to play games in Facebook Messenger
- Step 1: Open Facebook Messenger. This is a standalone app, completely separate from the standard Facebook app.
- Step 2: Find the games icon. Open a conversation, and tap the + sign in the bottom left.
- Step 3: Get gaming! Now all you need to do it pick a game and get playing.
- Step 4: Other ways to play.
Game Pigeon 20 Questions Answers Multiple Choice
How can I play iPhone games on my PC?
Launch iPadian, then you will see there is an iPad interface appearing on your PC. 3. Download a game or an app within iPadian's App Store, then you can play it on your PC exactly the same on your iPad/iPhone, except now you are using your mouse instead of fingers.
How do you fix pigeon on iMessage?
How to Fix iMessage effects not working in iOS 10
- Solution 1: Disable Reduce Motion.
- Step 1: Go to Settings -> General.
- Step 2: Open Accessibility and choose Reduce Motion.
- Step 3: If it's enabled, toggle it off.
- Solution 2: Disable iMessage & then turn on.
- Step 1: Launch the Settings app.
What are the best iMessage games?
8 Fun Games You Can Play Directly In iMessage
- Four in a Row (Free) Four in a Row is the iMessage adaptation of the classic game of Connect 4, and it's pretty fun.
- Fast Thumbs (Free) Fast Thumbs is a battle of speedy fingers.
- Cobi Hoops (Free) Cobi Hoops is a fairly ambitious looking basketball game.
- Mr. Putt (Free)
- MojiQuest (Free)
What games can you play over text?
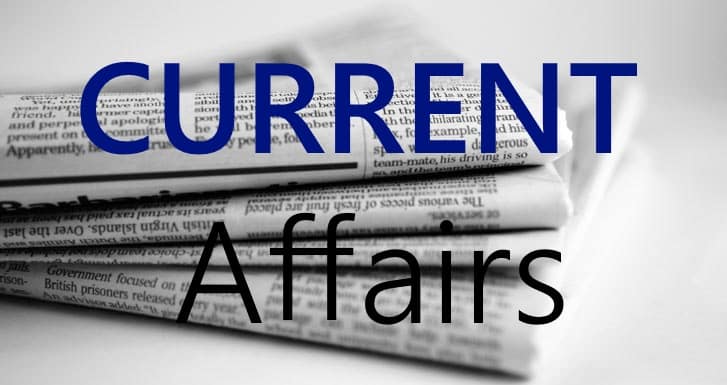
B. Background
As a child, you may have played a game called '20 questions', in which one player thinks of an animal, person, or object and the other person guesses the secret item by asking 20 questions. In this assignment you will be writing a modified version of this game. Your program will ask you to think of an animal, and then ask you up to N
yes or no questions in order to guess the animal you're thinking of. The questions and possible outcomes will be determined through a dataset we provide.
An online example of the game 20 Questions can be found at www.20q.net.
C. Your Program
First, download the skeleton code for GuessingGame.java
, the data sets animals_small.txt
and animals.txt
, and the library Prompter.java
for getting interactive input.
By the end of the assignment, your program GuessingGame
will (1) read in questions and answers data set from a file, (2) store the data in a 2D array, (3) sort the data so it can be easily searched, and (4) interact with the user to search for a target item. It will prompt the user with a number of questions until a single answer can be decided on. If there is no match the program should let the user know its been stumped.
The final product will look like:
1. Reading the Data
A. Data Format
Before you can start asking and answering questions, your program will need to have a large set of animals and some information about them. To do this, you'll read in a file in the following format:
animals_small.txt:
First, it provides the number of animals and questions in the data set. Then all the questions, each on their own line, and finally a list of animals and the answers to the questions ('y' is for yes; 'n' is for no). For instance, if you look at the line owl n y n
, the first column is the name of the animal (owl), the second is the answer to the first question (an owl is not a mammal), the third to the answer to the second question (owls are carnivores), and the last is the answer to the third question (owls do not stand on four legs). Once you feel comfortable with the format of the data, move on to the next section.
B. readData()
Now that you know what format the data will be in, you will write the function, readData(String filename)
, to parse through the animal.txt
file.
readData()
should be doing two things: 1.) initializing and populating a class-level array called String[] questions
, and 2.) initializing and populating a class-level 2D array String[][] answers
. Note that in the skeleton code we provided, readData()
has a void
return type, so you should not be returning either questions
or answers
.
After running readData('animals_small.txt')
, your arrays should contain:
Note that each row (the first index) in answers
corresponds to an animal, and each column (the second index) corresponds to an answer to a question (or the animal's name). Be careful -- it is very easy to mix this up. For reference, you can access a particular animal's attribute via answers[animalIndex][questionIndex]
, where animalIndex
and questionIndex
are the indices of the animal and question respectively (write this down somewhere!).
If you need a reminder how to read in from files, look back at previous homework assignments and read about In inStream
. Once you believe your readData()
is correct by eye, write the print()
function in the next section and then test your two functions in main()
.
C. print()
Being able to easily debug is essential when writing large programs. With this in mind, write a print()
function that outputs the content of the two class-level arrays.
print()
should print 1.) the number of animals, 2.) the number of questions, 3.) the questions
array, and 4.) the answers
array. The output should look EXACTLY like the file you read in, but you should construct this output based solely on the questions
and answers
arrays. Be sure that each animal has all its info on the same line in your output, and compare it to the original file.
To check whether your program is correct so far, write a short main()
function to first call readData('animals_small.txt')
and then print()
. Make sure that the output is identical to the content of the animals_small.txt
file. Once you can successfully do this, move on to the next section.
2. Sorting the Data
The next step is to sort the data set so that we can use standard searching techniques efficiently. However, unlike the sorting code we covered in class, we now have to deal with the 2D structure of the array AND the fact that we will be asking multiple questions.
Essentially, we need to reorder the rows (i.e., animals) so that each column is in sorted order, subject to row-order of the previous questions.
This complex idea should be easier to understand with an example. Imagine that we have the following small data set
We must first re-order the rows so that the first column is in sorted order:
This effectively divides the data set into two parts (blue and red), depending on the answer to the first question. Then, we have to re-order the rows WITHIN the blue and red blocks such that the 2nd column is in order:
Now, we effectively have four blocks, corresponding to the values of the first two attributes (nn, ny, yn, yy):
Next, we must sort the rows WITHIN each of these four blocks by the third column, yielding:
Notice that we reorder the rows WITHIN each block, but NEVER ACROSS blocks. We would then keep repeating this process for all subsequent attributes.
Read this example over several times until you thoroughly understand it. Once you do understand conceptually how this sort must work, move onto the next section.
B. Sorting in sort()
To do this sort, you will write several functions.
The first function you should write is:
public static void sortRange(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
This should sort the rows in the 2D array answers
from row indices startingAnimalIdx
to endingAnimalIdx
(not including endingAnimalIdx
) based on the column at index questionIdx
. For instance, if answers
looks like
and you called sortRange(0, 2, 2)
, you would be sorting the first two rows by the second column:
We can see that 'n' is earlier in the alphabet than 'y', and so these two rows should be switched, resulting in:
You are free to write this sorting function using either insertion sort or selection sort. You can look at the lecture materials to see a code implementation of either sort for 1D arrays, and you can base your code off the lecture code. However, not that your code will be substantially different from the lecture code since it must deal with the 2D structure of the array.
For comparing values, you should be using the String.compareTo()
function to compare the Strings. With Strings a
and b
, a.compareTo(b)
will return a negative number if a < b
, positive if a > b
, and zero if they are equal.
Once you can sort a column within a particular range of rows, you can move on to the next section. Write some code in main() to test your implementation. Remember, if you are having trouble debugging, use the print()
function to help figure out what is going wrong! Make sure it works correctly before moving onto the next part.
C. Finding the Split
We have one more helper function to write before we can do a complete sort through the data set. For every question, once we use sortRange()
to sort answers
by a question
, there will be an animal where everything above it answers 'n'
to question
and everything below has answers 'y'
. You will want to write the function
public static int findSplit(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
that will find the index of the first animal row that has the answer 'y'
to the question questionIdx
within the range startingAnimalIdx
to endingAnimalIdx
(not including endingAnimalIdx
). Note that this is a bit different than the standard binary search we discussed in class.
You should be comfortable with the intuition of binary search before you start coding!
Remember you will want to find the midpoint, and depending on the answer to the questionIdx
at that row, you will want to recurse either to the top or bottom half of the search range. You will need to check the value immediately below the midpoint in order to determine if it is the first 'y' to appear. If all of the answers in the range are 'y' or all are 'n', you'll want to return -1
.
Once you have this, you should be able to test it on animals_small.txt
. For example, findSplit(0, 4, 1)
should return 2.
If you are unable to get binary search working to find the split point, you may use linear search for partial credit.
D. Recursing in sort()
Once sortRange()
and findSplit()
are done, we then want to sort the entire answers
array, starting at the first question and then moving onto subsequent questions, exactly as in the example at the top of this section. This will be done recursively, which means writing a base case and recursive calls within the sorting code.
Write a recursive helper function public static void sort(int startingAnimalIdx, int endingAnimalIdx, int startingQuestionIdx)
to implement this sorting process. Note that this has a very similar signature to sortRange()
but behaves very differently in one respect -- this function sorts the given range of animals COMPLETELY, starting with the column index startingQuestionId
and moving onto all SUBSEQUENT columns. (In contrast, sortRange()
only sorts the given range for that particular column.)
Base Case: The base case is if the question
you are sorting by does not exist (i.e., you only have 3 questions but you are trying to sort based on the 4th question) OR if there is only one row remaining in the given range. In this case, you simply want to return without doing anything.
Recursive Calls: For each column, you'll want to make two recursive calls. Say, in animals_small.txt
you have split the group into mammals and non-mammals:
Next you want to look at only the non-mammals and split it by whether they are carnivores, then look only at the mammals and split by whether they are carnivores.
Following that intuition, we will want to do two recursive calls for every column, one for the 'n's, and one for the 'y's. So for each, you should find the top and bottom of the range to pass as the startingAnimalIdx
and endingAnimalIdx
arguments to sort()
(Note: this is where findSplit
comes in!). Remember to deal with the edge case where findSplit
returns -1
. Within the mammals, you don't want to sort by the mammals question again, so your question
argument shouldn't be the same, but should move to the next question (question + 1
).
Once you have finished and tested this recursive helper function, you should go back implement the sort()
function to recursively sort all columns of answers
. Think about what the initial range of the sort should be, and the first question that it should be sorted by.
3. Searching the Data
A. Narrowing the Possibilities
Now that we have our data ordered, we can quickly search through it. Consider the animals in animals_small.txt
:
Before your first question, all you know is that your search range is the entire list of animals. Once you ask the first question, and know that the animal is a mammal, your search range suddenly gets chopped in half:
This process is very similar to the binary search you talked about in lecture and implemented in findSplit
, although the split won't always be perfectly in half.
In order to make GuessingGame
interactive, we've provided the Prompter.java
library. It has only one function:
String prompt(String prefix)
If you call Prompter.prompt('> ')
it will print '> '
, wait for the user to enter a String
, and then returns that String
.
Once you feel comfortable with the conceptual side of this search, move on to write search()
.
B. search()
You now have all the pieces in place to begin guessing some animals! Similarly to sort()
, you will see there are two functions in your skeleton code called search
.
First, let's look at:
public static String search(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
This function should search through your data set, considering only the range from startingAnimalIdx
to endingAnimalIdx
, looking first at questionIdx
. As in other functions, animals[endingAnimalIdx]
is not included in the search range. It will be structured as a binary search. If you'd like a refresher, you should look back a the lecture material on binary search (there is even a code implementation there!).
search()
will be recursive, so you will need a base case and recursive calls:
Base Case: The base case is when there is only one element in your search range. Note that the answers[endingAnimalIdx]
row is not in the search range. You should return the single element's name (the actual name of the animal found in the zeroth column).
Function Body: You should System.out.println
the question
text and then use Prompter.prompt('> ')
to get the answer.
Recursive Call: First find the 'n' and 'y' split using findSplit
, then, if the user answered 'n', search from the startingAnimalIdx
of the range to where the split is. If the user answered 'y', search from the split to the endingAnimalIdx
of the range. You will also want to move on to the next question (question + 1
).
Once you have finished this function, complete:
public static String search()
It should be only one line long and call search(int startingAnimalIdx, int endingAnimalIdx, int questionIdx)
and search the entire list starting with the first question.
If this is done correctly, you should be able to play the game with the following code in your main()
:
4. Extra Credit
Game Pigeon 20 Questions Answers Questions And Answers
A. Create Your Own Dataset!
For extra credit, you can collect your own dataset to query using your program! Make sure it adheres to these rules:
- No two items should have the same set of answers. For instance, a bear and a lion are both carnivorous mammals that stand on four feet, so with the questions in
animals_small.txt
we wouldn't be able to differentiate them. - All answers should be 'yes' or 'no', and so all questions should be yes-no questions.
- The data set should contain at least 50 items
Before you submit, make sure your dataset works with your Program!
5. Submission
A. README
Complete readme_guessing.txt
in the same way you have done for previous assignments.
Game Pigeon 20 Questions Answers Answer
B. Submission
Submit GuessingGame.java
and readme_guessing.txt
on the course website. If you have completed extra credit please mention this in the readme, and submit it compressed as extra.zip
.
Before submission remove any print statements that were used for debugging or testing your functions.
Be sure that every method has an appropriate header comment, and that your code is well-documented.
To challenge your friends within iMessage:
Game Pigeon 20 Questions Cheats
- Enter a conversation with the person you want to play with.
- Tap the Apps icon located next to the iMessage text box.
- From the Apps menu, tap the Grid icon in the bottom-left of the screen.
- Scroll through your apps to find the game you want to play and tap it.
- Tap Create Game.
How do I get iMessage games on my iPhone?
Tap on '>' and tap on the App Store icon. Step 3. Tap the square dot icon and find the game you just installed in iMessage. Step 4.
How do you play games on iMessage?
Getting started with iMessage games is easy. First, bring up the conversation with your friend. Then select the App Store icon in the bar below the message box. That will bring up the iMessage App Store with games, stickers, and more for use only in the Messages app.
Can Android Play iMessage games?
The iMessages need to be sent through Apple's servers, and the only way to do this legitimately is to use an Apple device. Using an app running on a Mac computer as a server that relays messages to the Android device is a very smart way to make iMessage work on Android, where it is not technically supported.
How do you play games on iPhone?
Before you can play any game on the Messages app on your iPhone, you need to download the games from the App Store in Messages. To do that, follow these steps: 1.Go to your Home screen by pressing your iPhone's Home button. 2.From the Home screen, open your Messages app.
What are iMessage games?
There are three types of iMessage Apps you can install — games, apps, and stickers. You can access the iMessage App Store from the Messages app by tapping the App Store icon near the keyboard in a conversation. The list of stickers, games, and apps for iMessage are continuing to grow, and plenty more will come.
How do I get iMessage?
To use iMessage or FaceTime, you need to activate them on your iPhone, iPad, or iPod touch.
Turn off and restart iMessage and FaceTime
- Go to Settings > Messages and turn off iMessage.
- Go to Settings > FaceTime and turn off FaceTime.
- Restart your device.
- Turn iMessage and FaceTime back on.
Can Apple make iMessages on Android?
Apple May Make iMessage Work with Android (Report) Google already supports RCS in its Android Messages app, but so far only Sprint among the major U.S. carriers supports the protocol.
Is there an Android version of iMessage?
iMessage is so good that many smartphone users would love to see an Android version come out, although it's something Apple will probably never do. Android Messages, not to be confused with Hangouts or Allo, is Google's texting app, and a new version of the app will soon be available on your Android device.
Can you play iMessage games on iPad?
Open the Message App on your iPhone/iPad and enter a thread. Then tap on the App Store icon and you can visit App Store for iMessage to explore games on your device. In App Store, you can install games you want that are compatible to iMessage.
How do you play games on messenger?
How to play games in Facebook Messenger
- Step 1: Open Facebook Messenger. This is a standalone app, completely separate from the standard Facebook app.
- Step 2: Find the games icon. Open a conversation, and tap the + sign in the bottom left.
- Step 3: Get gaming! Now all you need to do it pick a game and get playing.
- Step 4: Other ways to play.
Game Pigeon 20 Questions Answers Multiple Choice
How can I play iPhone games on my PC?
Launch iPadian, then you will see there is an iPad interface appearing on your PC. 3. Download a game or an app within iPadian's App Store, then you can play it on your PC exactly the same on your iPad/iPhone, except now you are using your mouse instead of fingers.
How do you fix pigeon on iMessage?
How to Fix iMessage effects not working in iOS 10
- Solution 1: Disable Reduce Motion.
- Step 1: Go to Settings -> General.
- Step 2: Open Accessibility and choose Reduce Motion.
- Step 3: If it's enabled, toggle it off.
- Solution 2: Disable iMessage & then turn on.
- Step 1: Launch the Settings app.
What are the best iMessage games?
8 Fun Games You Can Play Directly In iMessage
- Four in a Row (Free) Four in a Row is the iMessage adaptation of the classic game of Connect 4, and it's pretty fun.
- Fast Thumbs (Free) Fast Thumbs is a battle of speedy fingers.
- Cobi Hoops (Free) Cobi Hoops is a fairly ambitious looking basketball game.
- Mr. Putt (Free)
- MojiQuest (Free)
What games can you play over text?
9 Fun Texting Games To Play For Couples
- 1 Kiss, Marry, Kill.
- 2 20 Questions.
- 3 Funny Picture Challenge.
- 4 Guess the Lyric/Line.
- 5 Name Trivia Challenge.
- 6 Truth or Dare.
- 7 Would you rather ….
- 8 Be your Muses.
How do you play 20 questions on iMessage?
After each guess, keep track of the number of guesses that are used until it reaches the limit of 20. Once 20 questions are used up, players may not ask any more questions. If a player correctly guesses the object before then, they become 'it' for the next game and choose the next person, place, or thing.
How do I activate iMessage with my phone number?
Go to Settings > Messages and make sure that iMessage is on. You might need to wait a moment for it to activate. Tap Send & Receive. If you see 'Use your Apple ID for iMessage,' tap it and sign in with the same Apple ID that you use on your Mac, iPad, and iPod touch.
How do I activate iMessage on my iPhone?
How to activate iMessage for iPhone or iPad
- Launch Settings From your home screen.
- Tap Messages.
- Tap the iMessage On/Off switch. The switch will be green when it's been turned on.
How do I enable iMessages on my iPhone 10?
So open the Settings app then scroll down until you find the Messages section. Tap on Messages and you'll see a new page with an option at the top to enable iMessage.
What is the difference between text message and iMessage?
If you're connected to Wi-Fi, you can send iMessages without using your cellular data or text messaging plan. iMessage is faster than SMS or MMS: SMS and MMS messages are sent using different technology than your iPhone uses to connect to the internet.
How do you delete iMessages on iOS 12?
1. Completely delete a sticker pack/iMessage application from your phone
- tap the App Store icon in the iMessage conversation view.
- tap the apps icon on the bottom left (4 rounded grey rectangles)
- long-tap the app you want to delete (they'll start wiggling)
- delete just like you'd delete any app on your phone.
- done.
How do I remove iMessage from my iPhone?
Game Pigeon 20 Questions Answers Questions
Complete these steps from your iPhone before starting to use your new smartphone:
- Launch Settings from the Home screen of your iPhone.
- Tap Messages.
- Tap the slider next to iMessage to turn it off.
- Go back to Settings.
- Tap on Facetime.
- Tap the slider next to Facetime to turn it off.
Photo in the article by 'Pexels' https://www.pexels.com/photo/restaurant-love-romantic-dinner-3044/
Related posts:
- How To Play Games On Ios 10 Messages?
- How To Play Imessage Games On Ios 10?
- Quick Answer: How To Play Games On Ios 10 Imessage?
- Quick Answer: How To Play Games On Imessage Ios 10?
- Quick Answer: How To Play Imessage Games On Android?
- Question: How To Play Ios 10 Games?